Social Login
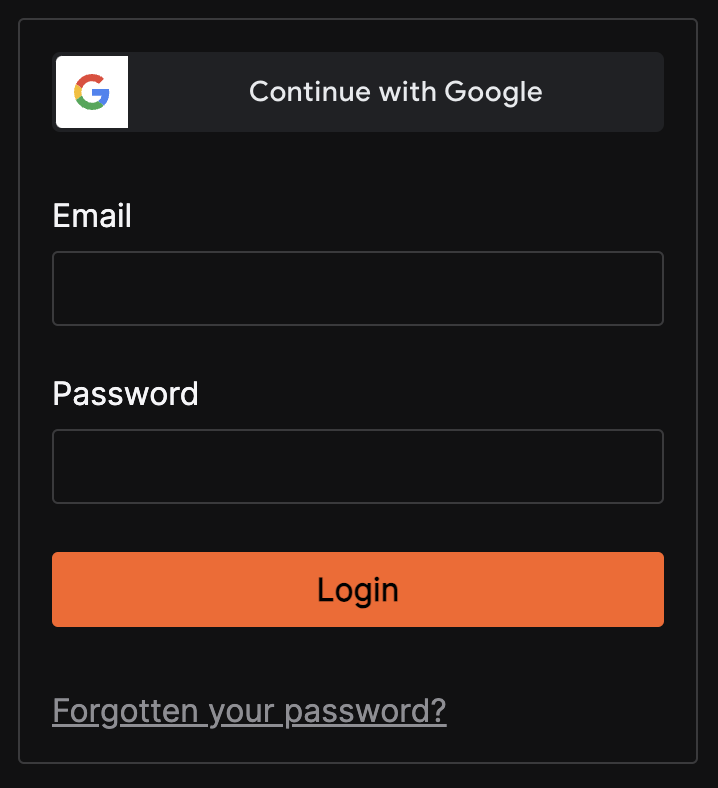
Marblism supports 40+ social login providers such as Github, Discord, Google, Facebook etc..
Google Login
Activation
Create your Google project and set your Client ID in your .env
file.
GOOGLE_CLIENT_ID=YOUR_CLIENT_ID
GOOGLE_CLIENT_SECRET=YOUR_CLIENT_SECRET
In the Google Dashboard
- set an Authorised JavaScript origins to
<your-web-url>
- set an Authorised redirect URIs to
<your-server-url>/api/auth/callback/google
.
Usage
The Login with Google
button appears automatically when your client ID is detected.
Social Login
All social logins work the same way, by defining a client id and secret in your .env
file and adding the social provider in src/core/authentication/index.ts
file. Let's see how to do it for Slack for example:
Activation
1. Add the SLACK_CLIENT_ID and SLACK_CLIENT_SECRET to your .env
file. For example:
SLACK_CLIENT_ID=YOUR_CLIENT_ID
SLACK_CLIENT_SECRET=YOUR_CLIENT_SECRET
2. Add the Slack provider to your authentication file
src/core/authentication/index.ts
import CredentialsProvider from 'next-auth/providers/credentials'
import GoogleProvider from 'next-auth/providers/google'
import SlackProvider from 'next-auth/providers/slack'
const providers: Provider[] = [
CredentialsProvider({
credentials: {
email: { type: 'email' },
password: { type: 'password' },
},
authorize: authorize(Database.getUnprotected()),
}),
GoogleProvider({
clientId: process.env.GOOGLE_CLIENT_ID as string,
clientSecret: process.env.GOOGLE_CLIENT_SECRET as string,
authorization: {
params: {
prompt: 'consent',
access_type: 'offline',
response_type: 'code',
},
},
}),
SlackProvider({
clientId: process.env.SLACK_CLIENT_ID,
clientSecret: process.env.SLACK_CLIENT_SECRET,
}),
/**
* ...add more providers here.
*/
]
Usage
The Login with Slack
button appears automatically in the login page.
Documentation
Some providers require a bit more work, check out the Next Auth documentation to see how to configure them.