Pusher
Marblism utilizes Pusher to seamlessly integrate WebSocket functionality. This allows you to create highly interactive and real-time applications, such as chat systems, live sports updates, and real-time collaboration tools, all benefiting from instantaneous communication between the client and server.
info
You must select the Socket (Pusher)
module during the project creation
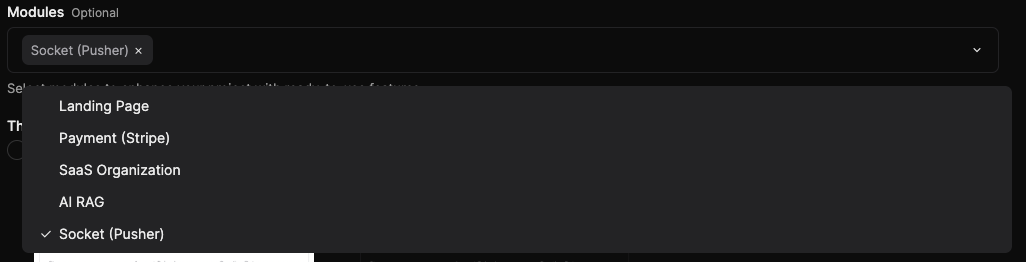
Activation
- Sign up with Pusher.
- Create an app in Channel.
- Select
React
for Front end. - Select
Node.js
for Back end.
- Select
- Head to the App Keys section.
- copy the secret keys.
- Add your environment variables to the your
.env
file:
PUSHER_APP_ID=
PUSHER_KEY=
PUSHER_SECRET=
PUSHER_CLUSTER=
PUBLIC_PUSHER_KEY=
PUBLIC_PUSHER_CLUSTER=
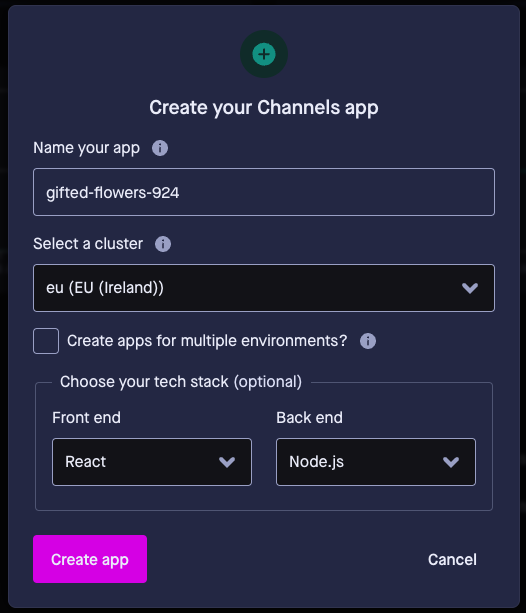
Usage
Use the Socket
api & usePusherClient
hooks in your front-end.
info
A Presence channel is implemented in the usePusherClient, which lets you know which users are connected.
import { Api } from '@/core/trpc'
import { usePusherClient } from '@/core/hooks/pusher'
export default function RoomListPage() {
const {pusherClient, connectedUserIds} = usePusherClient()
const { mutateAsync: emit } = Api.socket.emit.useMutation()
useEffect(() => {
if (!pusherClient) {
return
}
const channel = pusherClient.subscribe('private-room-join')
channel.bind('room-joined', data => {
// implement your logic here
})
return () => {
channel.unsubscribe('private-room-join')
}
}, [pusherClient])
const handleRoomClick = (roomId: string) => {
emit({
channel: 'private-room-join',
event: 'room-joined',
data: { userId: user.id, roomId, message: 'user joined on the room' },
})
}
...
}
Available endpoints
Api.socket.emit
Read More
Learn more on the official Pusher documentation