Analytics
Marblism integrates Posthog for web (visitors, referrering domains) and product (user actions, session recordings) analytics.
Activation
Posthog is activated by default.
Navigate to the tab Analytics
to see the number of visitors, page views, top page paths and referring domains.
app.marblism.com
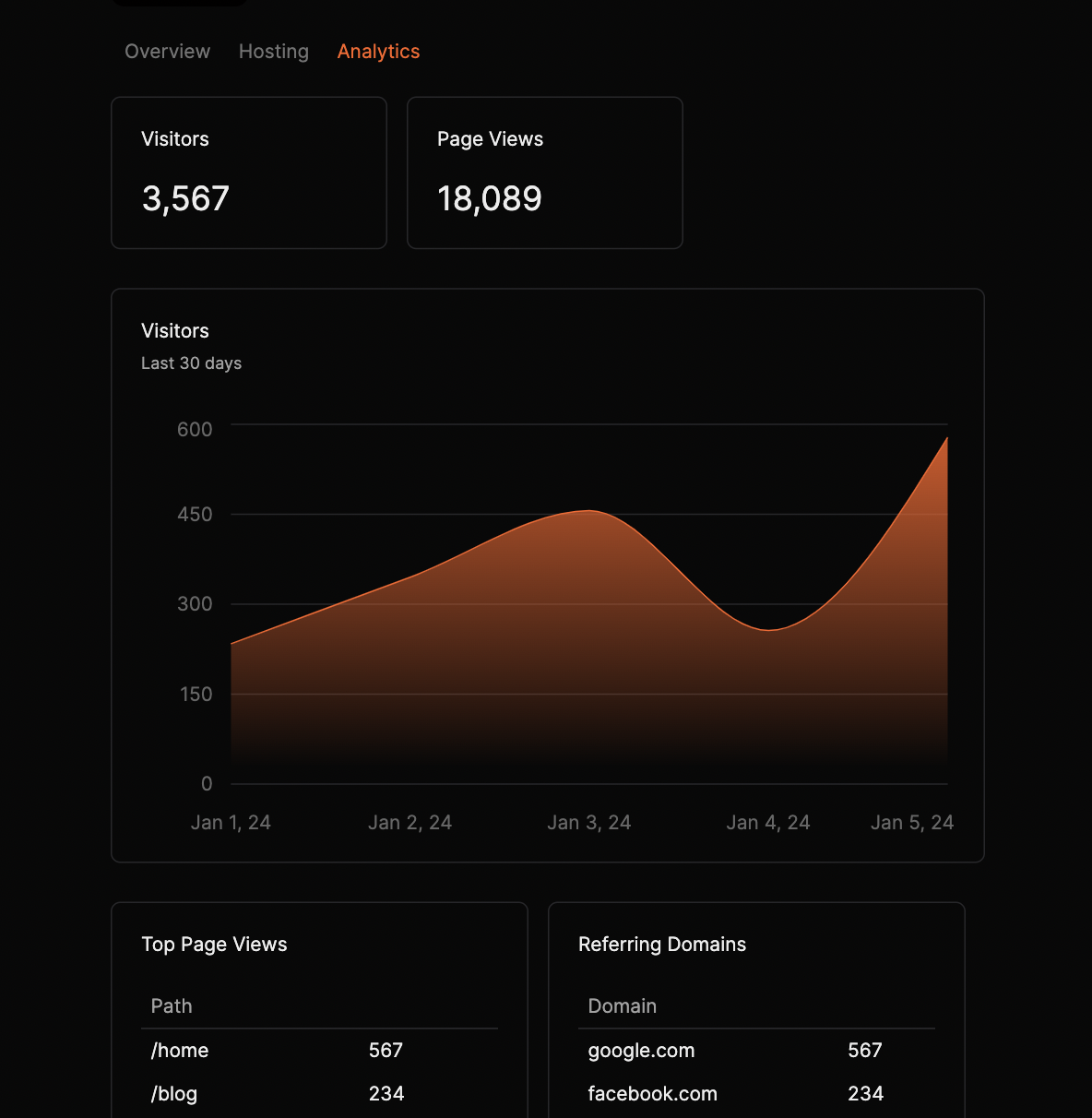
Use your own Posthog
Navigate to the tab Hosting
and set the following environment variables:
PUBLIC_POSTHOG_KEY=YOUR_PUBLIC_KEY
PUBLIC_POSTHOG_HOST=https://us.i.posthog.com
The analytics will then flow to your Posthog Dashboard.
Integration on old apps
If your app was created before the 10th of August 2024, you will have to add the integration on your codebase. Here is how to do it.
1). Install Posthog
pnpm add posthog-js
2). Create a file src/core/analytics/PostHogPageView.tsx
'use client'
import { usePathname, useSearchParams } from 'next/navigation'
import { usePostHog } from 'posthog-js/react'
import { useEffect } from 'react'
export default function PostHogPageView(): null {
const pathname = usePathname()
const searchParams = useSearchParams()
const posthog = usePostHog()
useEffect(() => {
// Track pageviews
if (pathname && posthog) {
let url = window.origin + pathname
if (searchParams.toString()) {
url = url + `?${searchParams.toString()}`
}
posthog.capture('$pageview', {
$current_url: url,
})
}
}, [pathname, searchParams, posthog])
return null
}
3). Create a file src/core/analytics/AnalyticsProvider.tsx
'use client'
import posthog from 'posthog-js'
import { PostHogProvider } from 'posthog-js/react'
import { useEffect } from 'react'
import { Api } from '../trpc'
export function AnalyticsProvider({ children }: { children: React.ReactNode }) {
const { data, isLoading } = Api.configuration.getPublic.useQuery()
useEffect(() => {
if (typeof window !== 'undefined' && !isLoading && data) {
const key = data['PUBLIC_POSTHOG_KEY']
const host = data['PUBLIC_POSTHOG_HOST']
posthog.init(key, {
api_host: host,
person_profiles: 'identified_only',
capture_pageview: false,
})
}
}, [data, isLoading])
return <PostHogProvider client={posthog}>{children}</PostHogProvider>
}
4). Update /src/app/layout.tsx
'use client'
import { AnalyticsProvider } from '@/core/analytics/AnalyticsProvider'
import dynamic from 'next/dynamic'
type Props = { children: React.ReactNode }
const PostHogPageView = dynamic(
() => import('../core/analytics/PostHogPageView'),
{
ssr: false,
},
)
export default function RootLayout({ children }: Props) {
return (
<DesignSystemProvider>
<SessionProvider>
<TRPCProvider>
<AnalyticsProvider> // <---- Add AnalyticsProvider here
<WorkspaceProvider>
<UserProvider>
<PostHogPageView /> // <---- Add PostHogPageView here
{children}
</UserProvider>
</WorkspaceProvider>
</AnalyticsProvider>
</TRPCProvider>
</SessionProvider>
</DesignSystemProvider>
)
}
5). Deploy your app.