Pages
In Remix, pages are defined following the file-based routing convention. This means that the file structure of your app/routes
directory directly corresponds to the routes of your application.
File Naming Convention
To target a page 'yourapp.com/my-route', the route can either be:
app/routes/my-route.tsx
app/routes/my-route/route.tsx
The first name inside the routes folder defines the URL. If it's a folder, it matches the route.tsx
file inside.
Dynamic routes
Let's say you want a user profile page about in your app. It will be:
app/routes/profile.$userId/route.tsx
It will be accessible from 'yourapp.com/profile/939ndn9393' '939ndn9393' being an example of a user id.
Layouts
Rather that re-defining your left bar, top navigation bar into every single pages, it's more efficient to define layouts that will always appear on your pages.
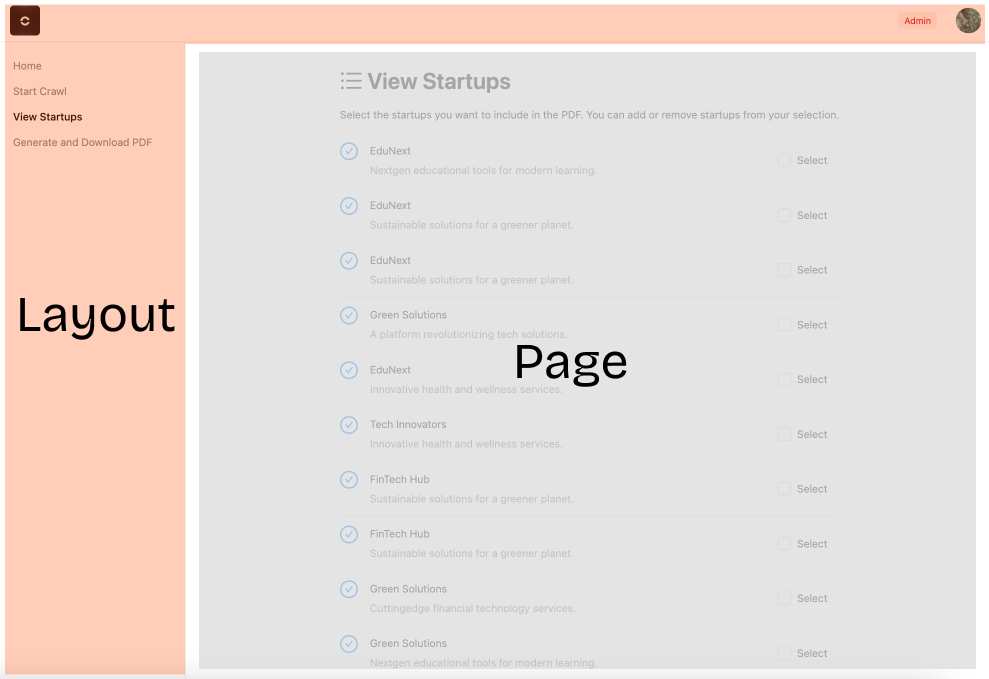
Here's an example of pages using a layout:
app/routes/_logged.users.tsx
app/routes/_logged.users_.$userId.tsx
These two files use the app/routes/_logged.tsx
layout where we define the navigation top bar ect..
Note the suffix on the users
segment. This is used when we don't want a page to act as a layout.
Here's a simple example of how these files might be structured:
// app/routes/_logged.tsx
export default function LoggedLayout({ children }) {
return (
<div>
<header>Logged In User</header>
<main>{children}</main>
</div>
)
}
// app/routes/_logged.users.tsx
export default function Users() {
return <h1>User List</h1>
}
// app/routes/_logged.users_.$userId.tsx
export default function UserDetail({ params }) {
return <h1>User Details for {params.userId}</h1>
}
In this example, _logged.tsx
defines a common layout for all logged-in pages. The users.tsx
file represents the user list page, and users_.$userId.tsx
represents individual user detail pages.
Page Prefixes
_logged
: Protected pages that require user authentication, display the navigation bar._auth
: Authentication pages (login, register, reset password)._public
: Public pages accessible by anybody.
For example:
app/routes/_logged.dashboard.tsx
: A protected dashboard pageapp/routes/_auth.login.tsx
: The login pageapp/routes/_public.about.tsx
: A public about page